가톨릭대학교 프로그래밍언어론 수업에서 제출한 자료 및 코드입니다.
프로그램 개요
학생 점수를 관리하는 프로그램입니다. 관리자가 학생들의 이름과 점수를 저장하면, 학생의 이름이나
번호를 통해서 해당 점수를 검색할 수 있습니다.
유즈케이스
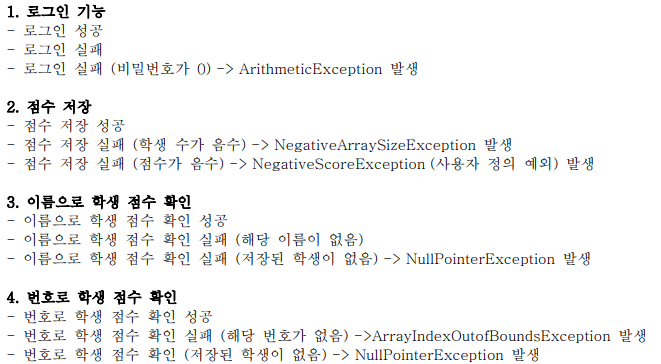
예외사항
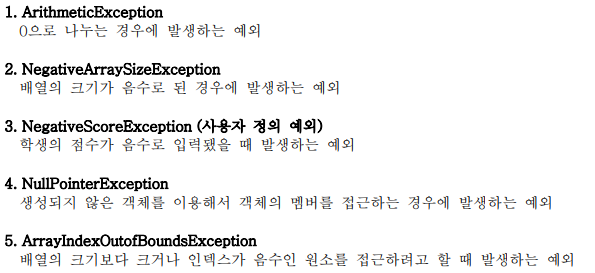
NullPointerException은 생성되지 않은 배열 객체의 원소를 접근하려는 경우에도 발생합니다.
클래스 다이어그램

커맨드 패턴을 사용하였습니다.
클래스 다이어그램 설명은 pdf를 이용해주시기 바랍니다.
코드
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
import java.util.ArrayList;
import java.util.Scanner;
// 사용자 정의 예외 (음수 점수 감지)
class NegativeScoreException extends Exception{
public NegativeScoreException(){
System.out.println("\n[ERROR] 점수는 음수일 수 없습니다! 초기화면으로 돌아갑니다!");
}
}
//해시 클래스
class SHA256 {
public String encrypt(String text) throws NoSuchAlgorithmException {
MessageDigest md = MessageDigest.getInstance("SHA-256");
md.update(text.getBytes());
return bytesToHex(md.digest());
}
private String bytesToHex(byte[] bytes) {
StringBuilder builder = new StringBuilder();
for (byte b : bytes) {
builder.append(String.format("%02x", b));
}
return builder.toString();
}
}
// 학생 점수 클래스
class student{
private String name;
private int score;
public student(String name, int score) {
this.name=name;
this.score=score;
}
public String getName() {
return name;
}
public int getScore() {
return score;
}
}
// 학생 점수 관리 클래스 (Receiver)
class studentManager{
private student students[];
private int student_count;
private Scanner sc=new Scanner(System.in);
private int num;
private String name;
private int score;
// 학생들 점수 저장
public void save() throws NegativeScoreException {
System.out.print("\n저장할 학생 수를 입력하시오:");
num=sc.nextInt();
try {
students=new student[num];
student_count=0;
for(int i=0; i<num; i++) {
System.out.print((i+1)+"번째 학생 이름을 입력하시오:");
name=sc.next();
System.out.print((i+1)+"번째 학생 점수를 입력하시오:");
score=sc.nextInt();
// 점수가 음수면 NegativeInputException
if(score<0) {
throw new NegativeScoreException();
}
else {
students[student_count++]=new student(name,score);
}
// 저장 완료
if(i==num-1) {
System.out.println("저장 완료!");
}
}
}
// 학생 수가 음수면 NegativeInputException
catch(NegativeArraySizeException e) {
System.out.println("\n[ERROR] 학생 수는 음수일 수 없습니다! 초기화면으로 돌아갑니다!");
}
}
// 이름으로 학생 점수 확인
public void findWithName() {
System.out.print("\n검색할 학생 이름을 입력하시오:");
name=sc.next();
try {
int i=0;
do {
student s=students[i];
String currentName=s.getName();
if(name.equals(currentName)) {
System.out.println(currentName+" 학생은 "+s.getScore()+"점입니다!\n");
break;
}
else if(i==students.length-1) {
System.out.println("존재하지 않는 학생입니다! 초기화면으로 돌아갑니다!");
}
i++;
}while(i<students.length);
}
// 저장된 학생이 없으면 NullPointerException
catch(NullPointerException e){
System.out.println("\n[ERROR] 저장된 학생이 없습니다! 초기화면으로 돌아갑니다!");
}
}
// 번호로 학생 점수 확인
public void findWithNumber() {
System.out.print("\n검색할 학생 번호를 입력하시오:");
num=sc.nextInt();
try {
student s=students[num-1];
System.out.println(s.getName()+" 학생은 "+s.getScore()+"점입니다!\n");
}
// 저장된 학생이 없으면 NullPointerException
catch(NullPointerException e){
System.out.println("\n[ERROR] 저장된 학생이 없습니다! 초기화면으로 돌아갑니다!");
}
// 존재하지 않는 번호면 ArrayIndexOutOfBoundsException
catch(ArrayIndexOutOfBoundsException e) {
System.out.println("\n[ERROR] 존재하지 않는 번호입니다! 초기화면으로 돌아갑니다!");
}
}
}
// 커맨드
interface Command{
public void execute();
}
// 저장 커맨드
class saveCommand implements Command{
private studentManager manager;
public saveCommand(studentManager manager) {
this.manager=manager;
}
public void execute(){
try { manager.save();}
catch (NegativeScoreException e) {}
}
}
// 이름으로 점수 확인 커맨드
class findScoreWithNameCommand implements Command{
private studentManager manager;
public findScoreWithNameCommand(studentManager manager) {
this.manager=manager;
}
public void execute(){
manager.findWithName();
}
}
// 번호로 점수 확인 커맨드
class findScoreWithNumberCommand implements Command{
private studentManager manager;
public findScoreWithNumberCommand(studentManager manager) {
this.manager=manager;
}
public void execute(){
manager.findWithNumber();
}
}
// 메뉴 클래스 (Invoker)
class ThreeButtonMenu{
private ArrayList<Command> commands=new ArrayList<>(3);
public void addCommand(Command command) {
commands.add(command);
}
public void button1pressed() {
commands.get(0).execute();
}
public void button2pressed() {
commands.get(1).execute();
}
public void button3pressed() {
commands.get(2).execute();
}
}
// 로그인 처리 클래스
class Login{
private int M=7;
private int id,pw;
private String answer="f5efb6e05e55f7eda6e88bec2eec69e6e2919cce997d7da9e6723e71b3021a87";
private SHA256 sha256 = new SHA256();
public Login(int id,int pw) {
this.id=id;
this.pw=pw;
}
public boolean process() throws NoSuchAlgorithmException {
try {
int C=1;
for(int i=0; i<id; i++) {
C=(C*M)%pw;
}
String output=sha256.encrypt(String.valueOf(C));
// 로그인 성공
if(answer.equals(output)) {
return true;
}
// 로그인 실패
else {
return false;
}
}
// 0으로 나누면 ArithmeticException
catch(ArithmeticException e){
System.out.println("\n[ERROR] 비밀번호는 0이 될 수 없습니다!");
return false;
}
}
}
public class admin {
public static void main(String[] args) throws NoSuchAlgorithmException{
// Invoker와 Receiver 객체 생성
ThreeButtonMenu menu=new ThreeButtonMenu();
studentManager mgr=new studentManager();
// 커맨드 설정
menu.addCommand(new saveCommand(mgr));
menu.addCommand(new findScoreWithNameCommand(mgr));
menu.addCommand(new findScoreWithNumberCommand(mgr));
// 로그인
System.out.println("== 로그인 ==");
Scanner sc=new Scanner(System.in);
System.out.print("\n* ID:");
int id=sc.nextInt();
System.out.print("* Password:");
int pw=sc.nextInt();
Login login=new Login(id,pw);
boolean connect=login.process();
// 로그인 성공 -> 점수 관리
while(connect) {
System.out.println("\n== 학생 점수 관리 프로그램 ==");
System.out.println("\n1. 점수 저장\n2. 이름으로 점수 확인\n3. 번호로 점수 확인\n");
System.out.print("* 선택해주세요:");
int select=sc.nextInt();
switch(select) {
case 1:
menu.button1pressed();
break;
case 2:
menu.button2pressed();
break;
case 3:
menu.button3pressed();
break;
default:
System.out.println("잘못된 입력입니다.");
break;
}
}
// 로그인 실패
System.out.println("\n로그인에 실패하였습니다!\n프로그램을 종료합니다!");
}
}
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
import java.util.ArrayList;
import java.util.Scanner;
// 사용자 정의 예외 (음수 점수 감지)
class NegativeScoreException extends Exception{
public NegativeScoreException(){
System.out.println("\n[ERROR] 점수는 음수일 수 없습니다! 초기화면으로 돌아갑니다!");
}
}
//해시 클래스
class SHA256 {
public String encrypt(String text) throws NoSuchAlgorithmException {
MessageDigest md = MessageDigest.getInstance("SHA-256");
md.update(text.getBytes());
return bytesToHex(md.digest());
}
private String bytesToHex(byte[] bytes) {
StringBuilder builder = new StringBuilder();
for (byte b : bytes) {
builder.append(String.format("%02x", b));
}
return builder.toString();
}
}
// 학생 점수 클래스
class student{
private String name;
private int score;
public student(String name, int score) {
this.name=name;
this.score=score;
}
public String getName() {
return name;
}
public int getScore() {
return score;
}
}
// 학생 점수 관리 클래스 (Receiver)
class studentManager{
private student students[];
private int student_count;
private Scanner sc=new Scanner(System.in);
private int num;
private String name;
private int score;
// 학생들 점수 저장
public void save() throws NegativeScoreException {
System.out.print("\n저장할 학생 수를 입력하시오:");
num=sc.nextInt();
try {
students=new student[num];
student_count=0;
for(int i=0; i<num; i++) {
System.out.print((i+1)+"번째 학생 이름을 입력하시오:");
name=sc.next();
System.out.print((i+1)+"번째 학생 점수를 입력하시오:");
score=sc.nextInt();
// 점수가 음수면 NegativeInputException
if(score<0) {
throw new NegativeScoreException();
}
else {
students[student_count++]=new student(name,score);
}
// 저장 완료
if(i==num-1) {
System.out.println("저장 완료!");
}
}
}
// 학생 수가 음수면 NegativeInputException
catch(NegativeArraySizeException e) {
System.out.println("\n[ERROR] 학생 수는 음수일 수 없습니다! 초기화면으로 돌아갑니다!");
}
}
// 이름으로 학생 점수 확인
public void findWithName() {
System.out.print("\n검색할 학생 이름을 입력하시오:");
name=sc.next();
try {
int i=0;
do {
student s=students[i];
String currentName=s.getName();
if(name.equals(currentName)) {
System.out.println(currentName+" 학생은 "+s.getScore()+"점입니다!\n");
break;
}
else if(i==students.length-1) {
System.out.println("존재하지 않는 학생입니다! 초기화면으로 돌아갑니다!");
}
i++;
}while(i<students.length);
}
// 저장된 학생이 없으면 NullPointerException
catch(NullPointerException e){
System.out.println("\n[ERROR] 저장된 학생이 없습니다! 초기화면으로 돌아갑니다!");
}
}
// 번호로 학생 점수 확인
public void findWithNumber() {
System.out.print("\n검색할 학생 번호를 입력하시오:");
num=sc.nextInt();
try {
student s=students[num-1];
System.out.println(s.getName()+" 학생은 "+s.getScore()+"점입니다!\n");
}
// 저장된 학생이 없으면 NullPointerException
catch(NullPointerException e){
System.out.println("\n[ERROR] 저장된 학생이 없습니다! 초기화면으로 돌아갑니다!");
}
// 존재하지 않는 번호면 ArrayIndexOutOfBoundsException
catch(ArrayIndexOutOfBoundsException e) {
System.out.println("\n[ERROR] 존재하지 않는 번호입니다! 초기화면으로 돌아갑니다!");
}
}
}
// 커맨드
interface Command{
public void execute();
}
// 저장 커맨드
class saveCommand implements Command{
private studentManager manager;
public saveCommand(studentManager manager) {
this.manager=manager;
}
public void execute(){
try { manager.save();}
catch (NegativeScoreException e) {}
}
}
// 이름으로 점수 확인 커맨드
class findScoreWithNameCommand implements Command{
private studentManager manager;
public findScoreWithNameCommand(studentManager manager) {
this.manager=manager;
}
public void execute(){
manager.findWithName();
}
}
// 번호로 점수 확인 커맨드
class findScoreWithNumberCommand implements Command{
private studentManager manager;
public findScoreWithNumberCommand(studentManager manager) {
this.manager=manager;
}
public void execute(){
manager.findWithNumber();
}
}
// 메뉴 클래스 (Invoker)
class ThreeButtonMenu{
private ArrayList<Command> commands=new ArrayList<>(3);
public void addCommand(Command command) {
commands.add(command);
}
public void button1pressed() {
commands.get(0).execute();
}
public void button2pressed() {
commands.get(1).execute();
}
public void button3pressed() {
commands.get(2).execute();
}
}
// 로그인 처리 클래스
class Login{
private int M=7;
private int id,pw;
private String answer="f5efb6e05e55f7eda6e88bec2eec69e6e2919cce997d7da9e6723e71b3021a87";
private SHA256 sha256 = new SHA256();
public Login(int id,int pw) {
this.id=id;
this.pw=pw;
}
public boolean process() throws NoSuchAlgorithmException {
try {
int C=1;
for(int i=0; i<id; i++) {
C=(C*M)%pw;
}
String output=sha256.encrypt(String.valueOf(C));
// 로그인 성공
if(answer.equals(output)) {
return true;
}
// 로그인 실패
else {
return false;
}
}
// 0으로 나누면 ArithmeticException
catch(ArithmeticException e){
System.out.println("\n[ERROR] 비밀번호는 0이 될 수 없습니다!");
return false;
}
}
}
public class admin {
public static void main(String[] args) throws NoSuchAlgorithmException{
// Invoker와 Receiver 객체 생성
ThreeButtonMenu menu=new ThreeButtonMenu();
studentManager mgr=new studentManager();
// 커맨드 설정
menu.addCommand(new saveCommand(mgr));
menu.addCommand(new findScoreWithNameCommand(mgr));
menu.addCommand(new findScoreWithNumberCommand(mgr));
// 로그인
System.out.println("== 로그인 ==");
Scanner sc=new Scanner(System.in);
System.out.print("\n* ID:");
int id=sc.nextInt();
System.out.print("* Password:");
int pw=sc.nextInt();
Login login=new Login(id,pw);
boolean connect=login.process();
// 로그인 성공 -> 점수 관리
while(connect) {
System.out.println("\n== 학생 점수 관리 프로그램 ==");
System.out.println("\n1. 점수 저장\n2. 이름으로 점수 확인\n3. 번호로 점수 확인\n");
System.out.print("* 선택해주세요:");
int select=sc.nextInt();
switch(select) {
case 1:
menu.button1pressed();
break;
case 2:
menu.button2pressed();
break;
case 3:
menu.button3pressed();
break;
default:
System.out.println("잘못된 입력입니다.");
break;
}
}
// 로그인 실패
System.out.println("\n로그인에 실패하였습니다!\n프로그램을 종료합니다!");
}
}
import java.security.MessageDigest; import java.security.NoSuchAlgorithmException; import java.util.ArrayList; import java.util.Scanner; // 사용자 정의 예외 (음수 점수 감지) class NegativeScoreException extends Exception{ public NegativeScoreException(){ System.out.println("\n[ERROR] 점수는 음수일 수 없습니다! 초기화면으로 돌아갑니다!"); } } //해시 클래스 class SHA256 { public String encrypt(String text) throws NoSuchAlgorithmException { MessageDigest md = MessageDigest.getInstance("SHA-256"); md.update(text.getBytes()); return bytesToHex(md.digest()); } private String bytesToHex(byte[] bytes) { StringBuilder builder = new StringBuilder(); for (byte b : bytes) { builder.append(String.format("%02x", b)); } return builder.toString(); } } // 학생 점수 클래스 class student{ private String name; private int score; public student(String name, int score) { this.name=name; this.score=score; } public String getName() { return name; } public int getScore() { return score; } } // 학생 점수 관리 클래스 (Receiver) class studentManager{ private student students[]; private int student_count; private Scanner sc=new Scanner(System.in); private int num; private String name; private int score; // 학생들 점수 저장 public void save() throws NegativeScoreException { System.out.print("\n저장할 학생 수를 입력하시오:"); num=sc.nextInt(); try { students=new student[num]; student_count=0; for(int i=0; i<num; i++) { System.out.print((i+1)+"번째 학생 이름을 입력하시오:"); name=sc.next(); System.out.print((i+1)+"번째 학생 점수를 입력하시오:"); score=sc.nextInt(); // 점수가 음수면 NegativeInputException if(score<0) { throw new NegativeScoreException(); } else { students[student_count++]=new student(name,score); } // 저장 완료 if(i==num-1) { System.out.println("저장 완료!"); } } } // 학생 수가 음수면 NegativeInputException catch(NegativeArraySizeException e) { System.out.println("\n[ERROR] 학생 수는 음수일 수 없습니다! 초기화면으로 돌아갑니다!"); } } // 이름으로 학생 점수 확인 public void findWithName() { System.out.print("\n검색할 학생 이름을 입력하시오:"); name=sc.next(); try { int i=0; do { student s=students[i]; String currentName=s.getName(); if(name.equals(currentName)) { System.out.println(currentName+" 학생은 "+s.getScore()+"점입니다!\n"); break; } else if(i==students.length-1) { System.out.println("존재하지 않는 학생입니다! 초기화면으로 돌아갑니다!"); } i++; }while(i<students.length); } // 저장된 학생이 없으면 NullPointerException catch(NullPointerException e){ System.out.println("\n[ERROR] 저장된 학생이 없습니다! 초기화면으로 돌아갑니다!"); } } // 번호로 학생 점수 확인 public void findWithNumber() { System.out.print("\n검색할 학생 번호를 입력하시오:"); num=sc.nextInt(); try { student s=students[num-1]; System.out.println(s.getName()+" 학생은 "+s.getScore()+"점입니다!\n"); } // 저장된 학생이 없으면 NullPointerException catch(NullPointerException e){ System.out.println("\n[ERROR] 저장된 학생이 없습니다! 초기화면으로 돌아갑니다!"); } // 존재하지 않는 번호면 ArrayIndexOutOfBoundsException catch(ArrayIndexOutOfBoundsException e) { System.out.println("\n[ERROR] 존재하지 않는 번호입니다! 초기화면으로 돌아갑니다!"); } } } // 커맨드 interface Command{ public void execute(); } // 저장 커맨드 class saveCommand implements Command{ private studentManager manager; public saveCommand(studentManager manager) { this.manager=manager; } public void execute(){ try { manager.save();} catch (NegativeScoreException e) {} } } // 이름으로 점수 확인 커맨드 class findScoreWithNameCommand implements Command{ private studentManager manager; public findScoreWithNameCommand(studentManager manager) { this.manager=manager; } public void execute(){ manager.findWithName(); } } // 번호로 점수 확인 커맨드 class findScoreWithNumberCommand implements Command{ private studentManager manager; public findScoreWithNumberCommand(studentManager manager) { this.manager=manager; } public void execute(){ manager.findWithNumber(); } } // 메뉴 클래스 (Invoker) class ThreeButtonMenu{ private ArrayList<Command> commands=new ArrayList<>(3); public void addCommand(Command command) { commands.add(command); } public void button1pressed() { commands.get(0).execute(); } public void button2pressed() { commands.get(1).execute(); } public void button3pressed() { commands.get(2).execute(); } } // 로그인 처리 클래스 class Login{ private int M=7; private int id,pw; private String answer="f5efb6e05e55f7eda6e88bec2eec69e6e2919cce997d7da9e6723e71b3021a87"; private SHA256 sha256 = new SHA256(); public Login(int id,int pw) { this.id=id; this.pw=pw; } public boolean process() throws NoSuchAlgorithmException { try { int C=1; for(int i=0; i<id; i++) { C=(C*M)%pw; } String output=sha256.encrypt(String.valueOf(C)); // 로그인 성공 if(answer.equals(output)) { return true; } // 로그인 실패 else { return false; } } // 0으로 나누면 ArithmeticException catch(ArithmeticException e){ System.out.println("\n[ERROR] 비밀번호는 0이 될 수 없습니다!"); return false; } } } public class admin { public static void main(String[] args) throws NoSuchAlgorithmException{ // Invoker와 Receiver 객체 생성 ThreeButtonMenu menu=new ThreeButtonMenu(); studentManager mgr=new studentManager(); // 커맨드 설정 menu.addCommand(new saveCommand(mgr)); menu.addCommand(new findScoreWithNameCommand(mgr)); menu.addCommand(new findScoreWithNumberCommand(mgr)); // 로그인 System.out.println("== 로그인 =="); Scanner sc=new Scanner(System.in); System.out.print("\n* ID:"); int id=sc.nextInt(); System.out.print("* Password:"); int pw=sc.nextInt(); Login login=new Login(id,pw); boolean connect=login.process(); // 로그인 성공 -> 점수 관리 while(connect) { System.out.println("\n== 학생 점수 관리 프로그램 =="); System.out.println("\n1. 점수 저장\n2. 이름으로 점수 확인\n3. 번호로 점수 확인\n"); System.out.print("* 선택해주세요:"); int select=sc.nextInt(); switch(select) { case 1: menu.button1pressed(); break; case 2: menu.button2pressed(); break; case 3: menu.button3pressed(); break; default: System.out.println("잘못된 입력입니다."); break; } } // 로그인 실패 System.out.println("\n로그인에 실패하였습니다!\n프로그램을 종료합니다!"); } }
정리 파일