STL: standard template library의 약자로서 많은 프로그래머들이 공통적으로 사용하는 자료구조와 알고리즘을 구현한 클래스들로 이루어져 있다. STL은 템플릿 기법을 사용하였기 때문에 어떤 자료형에 대해서도 사용할 수 있다.
템플릿: 다양한 자료형을 사용할 수 있도록 클래스를 설계하는 기법
(템플릿으로 작성된 클래스를 사용할 때는 원하는 자료형을 지정한다.)
ex) vector<배열의 자료형> 배열의 이름(배열의 크기);
1. 벡터 (Vector)
#include <iostream> #include <vector> #include <algorithm> #include <string> using namespace std; class Person { public: Person(string n, int a) :name(n), age(a) {} string get_name() const { return name; } int get_age() const { return age; } void print() { cout << name << " " << age << endl; } public: string name; int age; }; // 특정 멤버를 기준으로 오름차순 bool compare(Person& p, Person& q) { return p.get_age() < q.get_age(); } int main() { // 벡터 및 반복자 생성 vector<Person> v; vector<Person>::iterator it; // 벡터의 뒷 부분에서 추가 v.push_back(Person("Kim", 30)); v.push_back(Person("Park", 22)); v.push_back(Person("Lee", 26)); v.push_back(Person("Ahn", 23)); v.push_back(Person("Hyun", 19)); v.push_back(Person("Park", 22)); // 벡터의 특정 영역에 추가 // v.begin() -> 벡터의 시작 주소(첫 원소 주소) // v.end() -> 벡터의 끝 주소(마지막 원소에서 한 칸 더 간 주소) v.insert(v.begin(), Person("Kim2", 31)); v.insert(v.begin() + 1, Person("Kim3", 32)); v.insert(v.begin() + 2, Person("Kim4", 32)); // 벡터의 특정 영역 제거 v.erase(v.begin()); v.erase(v.begin(), v.begin() + 2); // begin~begin+1 // 벡터의 뒷 부분에서 제거 v.pop_back(); v.pop_back(); // 안정정렬 sort(v.begin(), v.end(), compare); // 벡터 원소 출력 (반복자 사용) for (it = v.begin(); it != v.end(); it++) it->print(); cout << endl; // 벡터 원소 출력 (범위 기반 for문) for (auto& e : v) e.print(); // 벡터의 모든 요소 삭제 if (!v.empty()) { cout <<"벡터 원소"<< v.size() <<"개 삭제"<<endl; v.clear(); } return 0; }
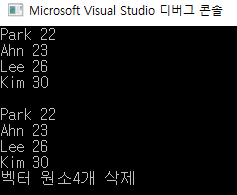
2. 스택 (Stack)
#include <iostream> #include <stack> using namespace std; int main() { stack<int> st; int arr[10] = { 1,2,3,4,5,6,7,8,9,10 }; // 삽입 for(auto& a : arr) st.push(a); // 삭제 while (!st.empty()) { cout << st.top() <<", size="<<st.size()<< endl; st.pop(); } return 0; }
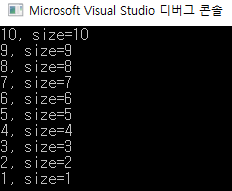
3. 큐 (Queue)
#include <iostream> #include <queue> using namespace std; int main() { queue<int> q; int arr[10] = { 1,2,3,4,5,6,7,8,9,10 }; // 삽입 for(auto& a : arr) q.push(a); // 삭제 while (!q.empty()) { cout << q.front() <<", size="<< q.size()<< endl; q.pop(); } return 0; }
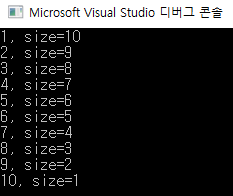
4-1. 우선순위 큐 (Max Heap)
#include <iostream> #include <queue> using namespace std; int main() { priority_queue<int> pq; int arr[10] = { 1,2,3,4,5,6,7,8,9,10 }; // 삽입 for(auto& a : arr) pq.push(a); // 삭제 while (!pq.empty()) { cout << pq.top() <<", size="<< pq.size()<< endl; pq.pop(); } return 0; }
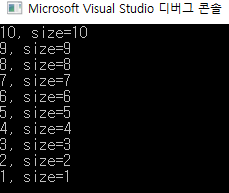
4-2. 우선순위 큐 (Min Heap)
#include <iostream> #include <queue> using namespace std; int main() { priority_queue<int, vector<int>,greater<int>> pq; int arr[10] = { 1,2,3,4,5,6,7,8,9,10 }; // 삽입 for(auto& a : arr) pq.push(a); // 삭제 while (!pq.empty()) { cout << pq.top() <<", size="<< pq.size()<< endl; pq.pop(); } return 0; }
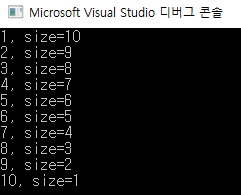
5. 덱 (Deque)
#include <iostream> #include <deque> using namespace std; int main() { deque<int> dq; int arr[10] = { 1,2,3,4,5,6,7,8,9,10 }; // 삽입 for (auto& a : arr) { if (a <= 5) dq.push_back(a); // 1 2 3 4 5 <- 후단 삽입 else dq.push_front(a); // 전단 삽입 -> 10 9 8 7 6 } // 10 9 8 7 6 1 2 3 4 5 // 후단 삭제 while (dq.size()>5) { cout << dq.back() <<", size="<< dq.size()<< endl; dq.pop_back(); } // 전단 삭제 while (!dq.empty()) { cout << dq.front() << ", size=" << dq.size() << endl; dq.pop_front(); } return 0; }
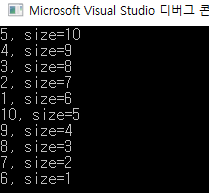
6. 집합 (Set)
#include <iostream> #include <set> using namespace std; int main() { set<int> s; s.insert(1); s.insert(2); s.insert(3); s.erase(2); // 집합 원소 못 찾으면 end() 반환 auto pos = s.find(2); if (pos != s.end()) cout << "find" << endl; else cout << "not find" << endl; return 0; }
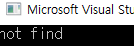
7. 맵 (Map)
#include <iostream> #include <string> #include <map> using namespace std; int main() { map<string, string> m; // 삽입 m["Ahn"] = "010-1234-5678"; m["Park"] = "010-2345-6789"; m["Ho"] = "010-3456-7890"; m.insert({"Kim","010-1212-3434"}); // 삭제 m.erase("Kim"); // 검색 if (m.find("Ho") == m.end()) cout << "not find" << endl; // 출력 for (auto& e : m) cout << e.first << ":" << e.second << endl; return 0; }
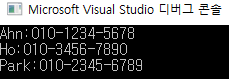