자료구조 c언어 스택 소스
#include <stdio.h> #define max 5 typedef struct { int top; int stack[max]; }stack_type; typedef stack_type* stack_type_ptr; void init(stack_type_ptr s) { s->top = -1; } int is_full(stack_type s) { if (s.top == max - 1) return 1; else return 0; } int is_empty(stack_type s) { if (s.top == -1) return 1; else return 0; } int pop(stack_type_ptr s) { if (!is_empty(*s)) { int tmp = s->stack[s->top--]; return tmp; } else printf("스택이 비어 있음"); } void push(stack_type_ptr s, int item) { if (!is_full(*s)) { s->top++; s->stack[s->top] = item; } else printf("스택이 꽉 참"); } int peek(stack_type s) { if (!is_full(s)) { int tmp = s.stack[s.top]; return tmp; } else printf("스택이 꽉 참"); } int main(void) { stack_type st; init(&st); push(&st, 10); // 10 ] push(&st, 11); // 11 10 ] push(&st, 12); // 12 11 10 ] printf("[ %d ]", pop(&st)); // 12 <- 11 10 ] printf("\n[ %d ]", peek(st)); // 11 10 ] }
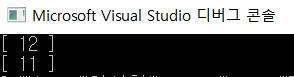