스택 사용해서 문자열 반대로 출력
#include <stdio.h> #define max 20 typedef struct { int stack[max]; int top; }stack_type; typedef stack_type* stack_type_ptr; stack_type st; void init(stack_type_ptr st) { st->top = -1; } int is_empty(stack_type st) { if (st.top == -1) return 1; else return 0; } int is_full(stack_type st) { if (st.top == max - 1) return 1; else return 0; } void push(stack_type_ptr st, int item) { if (!is_full(*st)) { st->top++; st->stack[st->top] = item; } else printf("스택이 전부 차 있음!!"); } int pop(stack_type_ptr st) { if (!is_empty(*st)) { char tmp = st->stack[st->top]; st->top--; return tmp; } } char get_symbol(char s[]) { static int i = -1; i++; return s[i]; } char reverse(char s[]) { char alpha = "0"; char tmp = 0; while (alpha != NULL) { alpha = get_symbol(s); push(&st, alpha); if (alpha == NULL) pop(&st); } while (!is_empty(st)) { tmp = pop(&st); printf("%c", tmp); } } int main() { char s[] = "abcdefg"; reverse(s); }
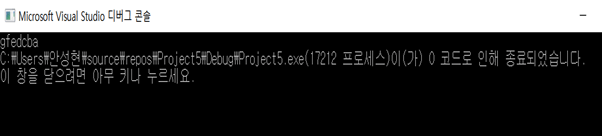