Union Array Tree Calculate
#include <stdio.h> typedef union { char op; int opnd; int is_thread; }element; void preorder(element *c, int i) { if (c[i].op != NULL) { if (c[i].op == '+' || c[i].op == '*' || c[i].op == '/') printf("%c ", c[i].op); else printf("%d ", c[i].opnd); preorder(c, 2 * i); preorder(c, 2 * i + 1); } } void inorder(element *c, int i) { if (c[i].op != NULL) { inorder(c, 2 * i); if (c[i].op == '+' || c[i].op == '*' || c[i].op == '/') printf("%c ", c[i].op); else printf("%d ", c[i].opnd); inorder(c, 2 * i + 1); } } void postorder(element *c, int i) { if (c[i].op != NULL) { postorder(c, 2 * i); postorder(c, 2 * i + 1); if (c[i].op == '+' || c[i].op == '*' || c[i].op == '/') printf("%c ", c[i].op); else printf("%d ", c[i].opnd); } } int power(int a, int b) { // 제곱 함수 if (b == 0) return 1; else return a * power(a, b - 1); } int calc(char operator, int leftopnd, int rightopnd) { switch (operator) { case '+': return leftopnd + rightopnd; case '-': return leftopnd - rightopnd; case '*': return leftopnd * rightopnd; case '/': return leftopnd / rightopnd; case '%': return leftopnd % rightopnd; case '^': return power(leftopnd, rightopnd); } } int eval(element *t, int i) { if (t[i].op == NULL) return 0; else if (t[2 * i].opnd == NULL && t[2 * i + 1].opnd == NULL) return t[i].opnd; else { int leftopnd = eval(t, 2 * i); int rightopnd = eval(t, 2 * i + 1); return calc(t[i].op, leftopnd, rightopnd); } } int get_count(element *t, int i) { int count = 0; if (t[i].op != 0) { count = 1 + get_count(t, 2 * i) + get_count(t, 2 * i + 1); } return count; } int main() { element c[16] = { 0,'+','*','/',3,2,30,6 }; printf("중위 표현: "); preorder(c, 1); printf("\n전위 표현: "); inorder(c, 1); printf("\n후위 표현: "); postorder(c, 1); printf("\n계산: %d", eval(c, 1)); printf("\n노드 개수: %d\n", get_count(c, 1)); system("pause"); }
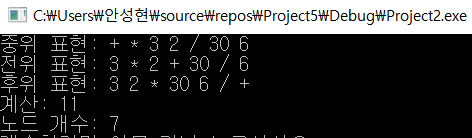