자료구조 – 노드(연결 리스트)로 구현된 리스트 c언어 소스
#include <stdio.h> #include <stdlib.h> typedef int element; typedef struct ListNode { element data; struct ListNode *link; }ListNode; ListNode* insert_first(ListNode *head, element value) { ListNode *p; p = (ListNode*)malloc(sizeof(ListNode)); p->data = value; p->link = head; head = p; return head; } // pre: 삽입할 노드의 이전 노드 ListNode* insert(ListNode *head, ListNode* pre, element value) { ListNode *p; p = (ListNode*)malloc(sizeof(ListNode)); p->data = value; p->link = pre->link; pre->link = p; return head; } ListNode* insert_last(ListNode *head, element value) { ListNode *p; p = head; while (p->link != NULL) { p = p->link; } p->link = (ListNode*)malloc(sizeof(ListNode)); p->link->data = value; p->link->link = NULL; return head; } ListNode* delete_first(ListNode *head) { ListNode *p = NULL; if (head != NULL) { p = head; head = p->link; } free(p); return head; } // pre: 삭제할 노드의 이전 노드 ListNode* delete(ListNode *head, ListNode *pre) { ListNode *p; p = pre->link; pre->link = p->link; free(p); return head; } ListNode* delete_last(ListNode *head) { ListNode *p; p = head; if (p->link == NULL) { free(p); head = NULL; } else { // 마지막에서 두 번째 노드의 link필드를 null로 만들기 while (p->link->link != NULL) { p = p->link; } free(p->link); p->link = NULL; } return head; } void print_list(ListNode* head) { ListNode *p = NULL; p = head; int i = 0; while (p != NULL) { printf("%d ", p->data); p = p->link; i++; } printf("(size:%d)\n", i); } int main() { ListNode* list = NULL; printf("삽입:"); list=insert_first(list,10); // 10 list = insert_first(list, 11); // 11 10 list = insert(list, list->link, 12); // 11 10 12 list = insert_last(list, 13); // 11 10 12 13 list = insert_last(list, 14); // 11 10 12 13 14 print_list(list); printf("\n삭제:"); list = delete_first(list); // 10 12 13 14 list = delete_last(list); // 10 12 13 list = delete(list, list->link); // 10 12 print_list(list); }
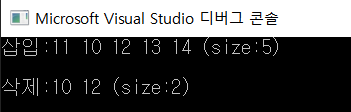