자료구조 – 배열로 구현한 리스트 c언어 소스
#include <stdio.h> #define max 100 typedef struct { int array[max]; int size; }ArrayListType; typedef ArrayListType * ArrayList_ptr; void init(ArrayList_ptr list) { list->size = 0; } int is_empty(ArrayListType list) { if (list.size == 0) return 1; else return 0; } int is_full(ArrayListType list) { if (list.size == max) return 1; else return 0; } void insert_first(ArrayList_ptr list, int item) { if (!is_full(*list)) { for (int i = list->size - 1; i >= 0; i--) list->array[i + 1] = list->array[i]; list->array[0] = item; list->size++; } else printf("리스트가 꽉 차있습니다\n"); } // 맨 처음에는 삽입 불가능한 함수 // list[pos] 공간에 삽입 // pos는 리스트의 현존하는 인덱스 -> 리스트 크기가 n이면 pos는 0~(n-1)까지 존재 void insert(ArrayList_ptr list, int pos, int item) { if (!is_full(*list) && pos >= 0 && pos <= list->size - 1) { for (int i = list->size - 1; i >= pos; i--) list->array[i + 1] = list->array[i]; list->array[pos] = item; list->size++; } else printf("리스트가 꽉 차있습니다\n"); } void insert_last(ArrayList_ptr list, int item) { if (!is_full(*list)) { list->array[list->size] = item; list->size++; } else printf("리스트가 꽉 차있습니다\n"); } int delete_first(ArrayList_ptr list) { if (!is_empty(*list)) { int tmp = list->array[0]; for (int i = 1; i < list->size; i++) list->array[i - 1] = list->array[i]; list->size--; return tmp; } else printf("리스트가 비어 있습니다\n"); } int delete(ArrayList_ptr list, int pos) { if (!is_empty(*list) && pos >= 0 && pos < list->size) { int tmp = list->array[pos]; for (int i = pos + 1; i < list->size; i++) list->array[i - 1] = list->array[i]; list->size--; return tmp; } else printf("리스트가 비어 있습니다\n"); } int delete_last(ArrayList_ptr list) { if (!is_empty(*list)) { int tmp = list->array[list->size - 1]; list->size--; return tmp; } else printf("리스트가 비어 있습니다\n"); } void print_list(ArrayListType list) { for (int i = 0; i < list.size; i++) printf("%d ", list.array[i]); printf("size:%d\n", list.size); } int main() { ArrayListType list; init(&list); printf("삽입:"); insert_first(&list, 10); // 10 insert_first(&list, 11); // 11 10 insert_last(&list, 12); // 11 10 12 insert_last(&list, 13); // 11 10 12 13 insert(&list, 3,144); // 11 10 12 144 13 print_list(list); printf("\n삭제:"); delete_first(&list); // 10 12 144 13 delete_last(&list); // 10 12 144 delete(&list, 1); // 10 144 print_list(list); }
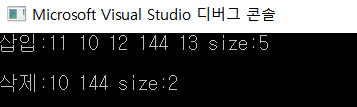
실용적인 리스트 소스 (Last In Last Out 구조 + 인덱스로 삭제)
#include <stdio.h> #include <stdlib.h> #pragma warning(disable:4996) #define max 30 typedef int element; typedef struct { element array[max]; int size; }ArrayListType; typedef ArrayListType * ArrayList_ptr; // 리스트 초기화 void init(ArrayList_ptr list) { list->size = 0; } // 리스트 생성 ArrayList_ptr create() { return (ArrayList_ptr)calloc(1, sizeof(ArrayListType)); } // 빈 리스트 확인 int is_empty(ArrayList_ptr list) { if (list->size == 0) return 1; else return 0; } // 풀 리스트 확인 int is_full(ArrayList_ptr list) { if (list->size == max) return 1; else return 0; } // 아이템 삽입 void insert_last(ArrayList_ptr list, element item) { if (!is_full(list)) { int pos = list->size; list->array[pos] = item; list->size++; } else printf("리스트가 꽉 차있습니다\n"); } // 아이템 삭제 void delete_last(ArrayList_ptr list) { if (!is_empty(list)) { list->size--; } else printf("리스트가 비어 있습니다\n"); } // 인덱스로 아이템 삭제 void delete_idx(ArrayList_ptr list, int pos) { if (!is_empty(list)) { element tmp = list->array[pos]; for (int i = pos + 1; i < list->size; i++) list->array[i - 1] = list->array[i]; list->size--; } else printf("리스트가 비어 있습니다\n"); } // 리스트 출력 void print_list(ArrayList_ptr list) { if (!is_empty(list)) { for (int i = 0; i < list->size; i++) printf("[%d] ", list->array[i]); } else printf("리스트가 비어 있습니다\n"); } int main() { ArrayList_ptr list = create(); init(list); insert_last(list, 1); // [1] insert_last(list, 2); // [1 2] insert_last(list, 3); // [1 2 3] insert_last(list, 4); // [1 2 3 4] insert_last(list, 5); // [1 2 3 4 5] delete_last(list); // [1 2 3 4] delete_last(list); // [1 2 3] delete_idx(list, 0); // [2 3] insert_last(list, 4); // [2 3 4] delete_idx(list, 1); // [2 4] print_list(list); // 리스트 요소 출력 }