BMI Calculator 에 들어간 클래스/위젯
class, component on the BMI Calculator
- Intetent for moving Activity
- Sharedpreference
- EditText , Button
- ImageView
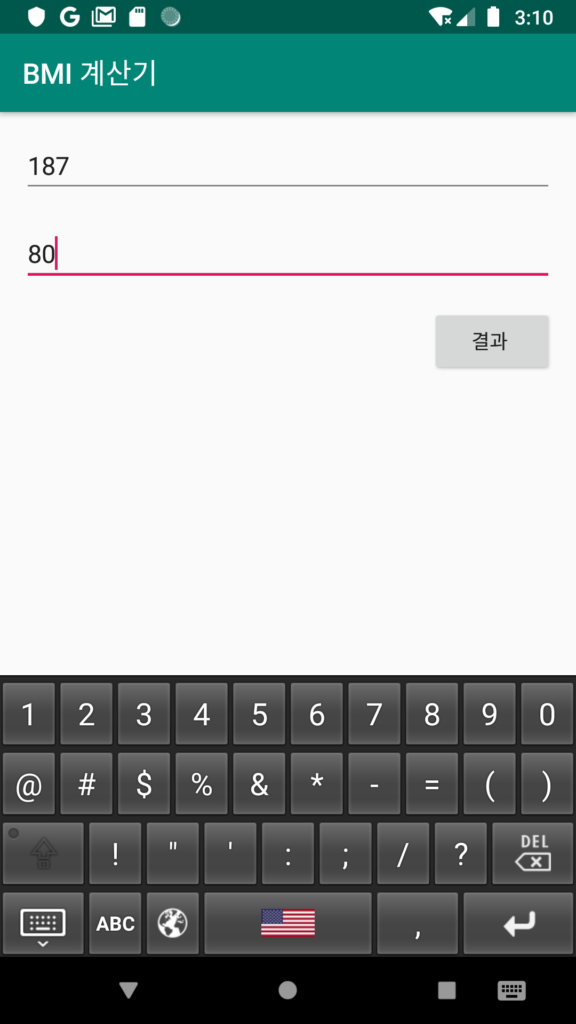
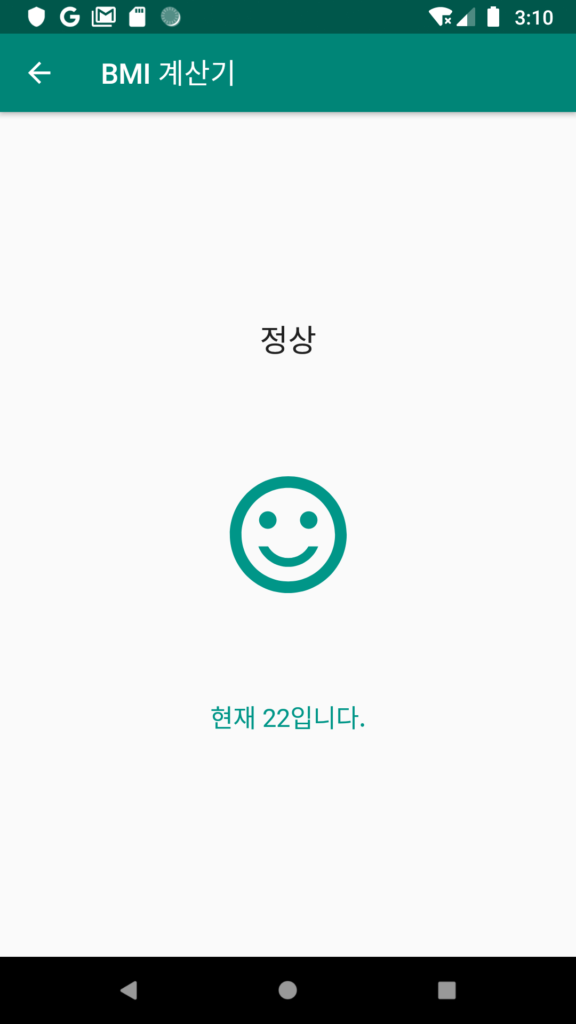
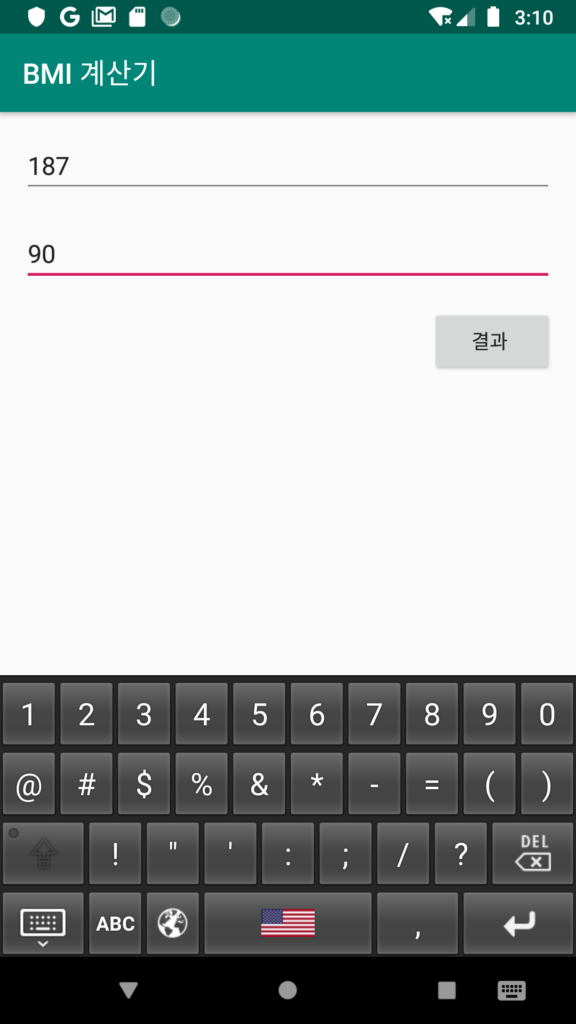
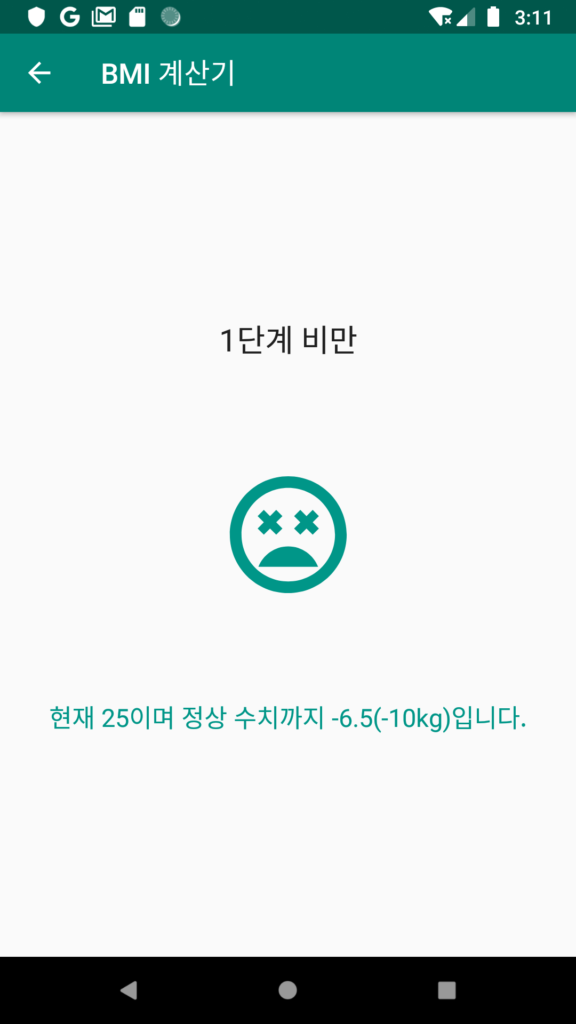
It has 2 Activities =>
- activity_main.xml
- MainActivity.kt
- activity_result.xml
- ResultActivity.kt
I used anko library for convinience.
activity_main.xml
<?xml version="1.0" encoding="utf-8"?> <androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" xmlns:app="http://schemas.android.com/apk/res-auto" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity"> <EditText android:layout_width="0dp" android:layout_height="wrap_content" android:inputType="number|textPersonName" android:ems="10" android:id="@+id/heightEditText" app:layout_constraintStart_toStartOf="parent" android:layout_marginLeft="16dp" android:layout_marginStart="16dp" app:layout_constraintEnd_toEndOf="parent" android:layout_marginEnd="16dp" android:layout_marginRight="16dp" android:hint="@string/height" app:layout_constraintHorizontal_bias="0.0" app:layout_constraintTop_toTopOf="parent" android:layout_marginTop="16dp"/> <EditText android:layout_width="0dp" android:layout_height="wrap_content" android:inputType="number|textPersonName" android:ems="10" android:id="@+id/weightEditText" app:layout_constraintStart_toStartOf="parent" android:layout_marginLeft="16dp" android:layout_marginStart="16dp" app:layout_constraintEnd_toEndOf="parent" android:layout_marginEnd="16dp" android:layout_marginRight="16dp" android:hint="몸무게" android:layout_marginTop="18dp" app:layout_constraintTop_toBottomOf="@+id/heightEditText"/> <Button android:text="결과 " android:layout_width="wrap_content" android:layout_height="wrap_content" android:id="@+id/resultButton" android:layout_marginTop="16dp" app:layout_constraintTop_toBottomOf="@+id/weightEditText" app:layout_constraintEnd_toEndOf="parent" android:layout_marginEnd="16dp" android:layout_marginRight="16dp"/> </androidx.constraintlayout.widget.ConstraintLayout>
MainActivity.kt
package com.example.myapplication import android.os.Bundle import androidx.appcompat.app.AppCompatActivity import kotlinx.android.synthetic.main.activity_main.* import org.jetbrains.anko.startActivity import org.jetbrains.anko.toast class MainActivity : AppCompatActivity() { /* 쉐어드 프리퍼런스 저장 메소드: 쉐어드 프리퍼런스는 App에 포함되는 데이터 파일을 만들어서 디바이스에 저장하거나 관리하는 객체이다. 데이터 파일을 편집할 때는 에디터 객체를 불러와야 한다. (덕분에 클래스 외부로 데이터를 보내거나, 외부 데이터를 불러올 수 있음) */ private fun saveData(height:String,weight:String){ val pref = this.getPreferences(0) val editor=pref.edit() // 키와 밸류를 쌍으로 저장하고 apply한다 editor.putString("KEY_HEIGHT",height) .putString("KEY_WEIGHT",weight) .apply() } // 쉐어드 프리퍼런스 로드 메소드 private fun loadData(){ val pref=this.getPreferences(0) // 키에 해당되는 밸류를 가져오는데 저장된 값이 없으면 ""을 가져온다 val height=pref.getString("KEY_HEIGHT","") val weight=pref.getString("KEY_WEIGHT","") if(!height.equals("") && !height.equals("")){ heightEditText.setText(height.toString()) weightEditText.setText(weight.toString()) } } override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) // 쉐어드 프리퍼런스 이용해서 editText 데이터 세팅 loadData() resultButton.setOnClickListener{ // editText데이터 불러와서 정수형으로 만들고 저장 val w:String = weightEditText.text.toString() val h:String = heightEditText.text.toString() // 값을 가져 왔는데 비어있지 않을 때 if문 성립 if(!w.equals("") && !h.equals("")){ // 쉐어드 프리퍼런스 이용해서 영구적으로 데이터 저장 saveData(h,w) // anko라이브러리 사용해서 액티비티 이동 및 인텐트에 데이터 저장 쉽게 해결 startActivity<ResultActivity>("weight" to w.toInt(), "height" to h.toInt())} else toast("키와 몸무게를 입력해주세요!") } } }
activity_result.xml
<?xml version="1.0" encoding="utf-8"?> <androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" xmlns:app="http://schemas.android.com/apk/res-auto" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".ResultActivity"> <TextView android:text="결과가 표시되는 곳" android:layout_width="wrap_content" android:layout_height="wrap_content" android:id="@+id/resultTextView" app:layout_constraintStart_toStartOf="parent" android:layout_marginLeft="16dp" android:layout_marginStart="16dp" app:layout_constraintEnd_toEndOf="parent" android:layout_marginEnd="16dp" android:layout_marginRight="16dp" android:layout_marginTop="130dp" app:layout_constraintTop_toTopOf="parent" android:textAppearance="@style/TextAppearance.AppCompat.Large" android:layout_marginBottom="57dp" app:layout_constraintBottom_toTopOf="@+id/imageView"/> <ImageView android:layout_width="100dp" android:layout_height="100dp" app:srcCompat="@drawable/ic_sentiment_satisfied_black_24dp" android:id="@+id/imageView" android:tint="#009688" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintStart_toStartOf="parent" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintTop_toTopOf="parent"/> <TextView android:text="설명이 표시되는 곳" android:layout_width="wrap_content" android:layout_height="wrap_content" android:id="@+id/description" app:layout_constraintStart_toStartOf="parent" android:layout_marginLeft="16dp" android:layout_marginStart="16dp" app:layout_constraintEnd_toEndOf="parent" android:layout_marginEnd="16dp" android:layout_marginRight="16dp" android:textAppearance="@style/TextAppearance.AppCompat.Medium" android:textColor="#009688" android:layout_marginTop="68dp" app:layout_constraintTop_toBottomOf="@+id/imageView"/> </androidx.constraintlayout.widget.ConstraintLayout>
ResultActivity.kt
package com.example.myapplication import android.os.Bundle import androidx.appcompat.app.AppCompatActivity import kotlinx.android.synthetic.main.activity_result.* class ResultActivity : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_result) // getStringExtra 메소드를 사용해서 인텐트에 저장된 정수 데이터를 가져 온다. val height=intent.getIntExtra("height",0) val weight=intent.getIntExtra("weight",0) // BMI 계산 val bmi:Int =(weight/Math.pow(height/100.0, 2.0)).toInt() // height기준으로 정상 체중 계산 val normalw:Int =(22*Math.pow(height/100.0, 2.0)).toInt() // 결과 표시 when{ bmi >= 35-> resultTextView.text="고도 비만" bmi >= 30-> resultTextView.text="2단계 비만" bmi >= 25-> resultTextView.text="1단계 비만" bmi >= 23-> resultTextView.text="과체중" bmi >=18.5-> resultTextView.text="정상" else -> resultTextView.text="저체중" } // 이미지 및 텍스트 표시 when{ bmi>=23->{ imageView.setImageResource(R.drawable.ic_sentiment_very_dissatisfied_black_24dp) description.setText("현재 ${bmi}이며 정상 수치까지 -${bmi-22}(-${weight-normalw}kg)입니다.")} bmi>=18.5->{ imageView.setImageResource(R.drawable.ic_sentiment_satisfied_black_24dp) description.setText("현재 ${bmi}입니다.")} else->{ imageView.setImageResource(R.drawable.ic_sentiment_dissatisfied_black_24dp) description.setText("현재 ${bmi}이며 정상 수치까지 +${22-bmi}(+${normalw-weight}kg)입니다.")} } } }